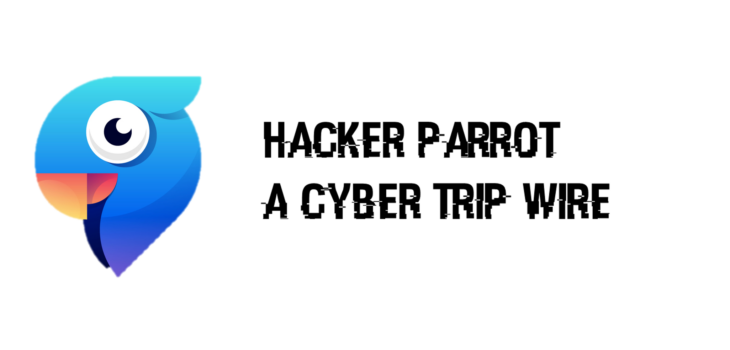
Ok About 4 months ago I made a quick PHP page to be used as a custom ALARM for detecting intruders in a network. This has a quick call to a Slack Web Hook but clearly could link to anything (.e.g. DISCORD, TEAMS etc.)
Today I wanted to deploy this but I thought I’d throw it into CHATGPT to see how it summarizes code:
So here we go:
This appears to be a simple HTML page with PHP code embedded in it. The PHP code retrieves the IP address and user agent of the client accessing the page and sends this information, along with the IP address of the server hosting the PHP code, to a Slack channel using a webhook.
The PHP code starts by retrieving the IP address and user agent of the client accessing the page using the $_SERVER superglobal variables. It then uses the curl library to retrieve the IP address of the server hosting the PHP code from the URL https://ip.me. The retrieved IP address of the server and the IP address and user agent of the client are then combined into a message that is sent to a Slack channel using a webhook.
The Slack webhook URL is defined as a constant using the define() function, and the message to be sent is defined as an array containing a payload key with a value of a JSON-encoded array containing a text key with the message to be sent. The curl library is used to send the message to the Slack webhook URL. The SSL verification option is disabled using CURLOPT_SSL_VERIFYPEER to avoid SSL certificate validation errors. The message is sent as a POST request with the message data set as the request body. Finally, the PHP code outputs the text “Complete” to the HTML page, indicating that the process has finished.
CHATGPT
The code is here:
<html>
<head>
<title>Logon Page</title>
</head>
<body>
Loading...
<?php
$cip = $_SERVER['REMOTE_ADDR'];
$agent = $_SERVER['HTTP_USER_AGENT'];
echo $cip;
define('MY_IP', 'https://ip.me');
$myip = curl_init(MY_IP);
curl_setopt($myip, CURLOPT_RETURNTRANSFER, true);
$ip = curl_exec($myip);
echo($ip);
// Create a constant to store your Slack URL
define('SLACK_WEBHOOK', 'https://hooks.slack.com/services/WEBOOOK');
// Slack Message
$txtstr = ('Canary from pi: ');
$txt = ($txtstr . strval($ip) . ' Client IP:' . $cip . ' USER AGENT: ' . $agent);
$message = array('payload' => json_encode(array('text' => $txt)));
// Use curl
$c = curl_init(SLACK_WEBHOOK);
curl_setopt($c, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($c, CURLOPT_POST, true);
curl_setopt($c, CURLOPT_POSTFIELDS, $message);
curl_exec($c);
curl_close($c);
echo 'Complete';
?>
</body>
</html>
I’m going to see now if we can get chatGPT to write another version….
https://github.com/mr-r3b00t/parrot
Ok so I have asked for a php snippet that will send a teams message via webhook:
<?php
// Define the message to be sent to Microsoft Teams
$message = array(
'text' => 'Hello, this is a message from PHP!'
);
// Encode the message as JSON
$message_json = json_encode($message);
// Define the Incoming Webhook URL provided by Microsoft Teams
$webhook_url = 'https://outlook.office.com/webhook/XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX';
// Set up the HTTP request
$options = array(
'http' => array(
'header' => "Content-Type: application/json\r\n",
'method' => 'POST',
'content' => $message_json,
),
);
// Send the HTTP request to the Incoming Webhook URL
$context = stream_context_create($options);
$result = file_get_contents($webhook_url, false, $context);
// Output the result of the request
echo $result;
?>
I’m going to deploy and see if we can have some fun! Now these are basic, you can clearly get very very creative with how to stand up deception and detection technology inside a network.
OK quick update, I asked CHATGPT to make a teams webhook php page which sent the source IP, Public IP and UserAgent and we got the following configured in teams in minutes:
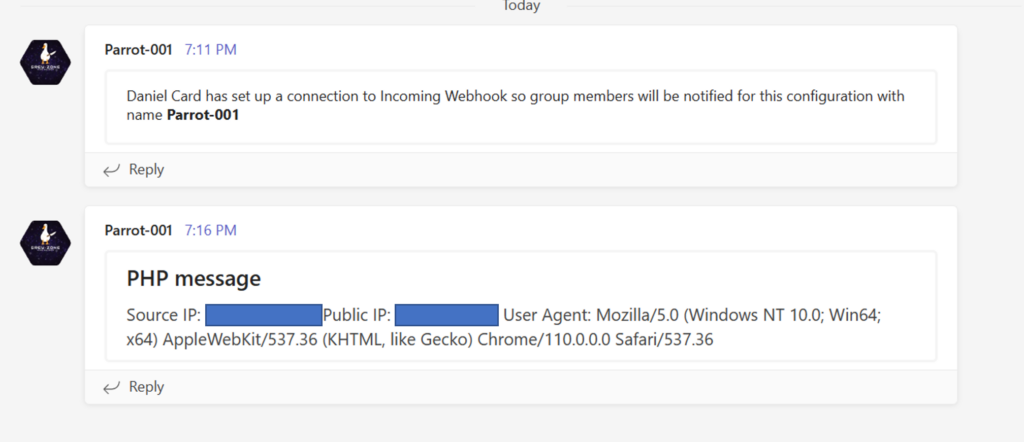
That’s quite neat!
My PoC code + CHATGPT snippets are here:
https://github.com/mr-r3b00t/parrot
Hopefully this is useful, you might also want to think about x-forwarded for headers etc. it depends on the use case obviously.