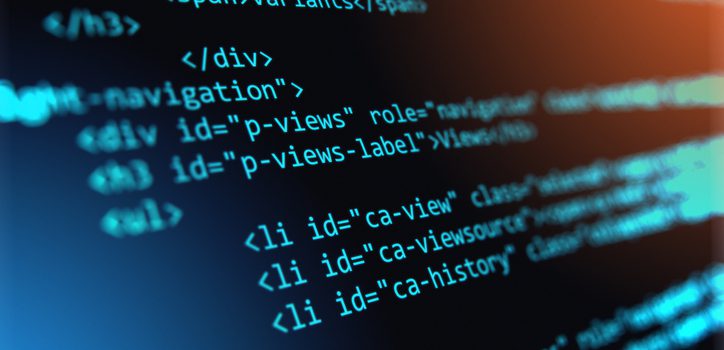
Today’s tip is a quick post on how to create self signed HTTPS web services in python for when you need to transfer a file fast! Now in a live environment you are likely going to need to use a CA signed service such as LetsEncrypt etc. otherwise your clients will get a warning (or they will just click Accept and Continue etc. as most people do! However this is a quick post to show how to use Python3 to host http and https services for staging payloads etc.
Python HTTP Servers
Now in the python 2.7 days creating a web server was quite simple:
python -m SimpleHTTPServer 80
Now with python3 you need to be using the following:
python3 -m http.server
If you need a fast https server to host a payload then here’s a quick script to get you moving!
First we need to generate a certificate:
openssl req -new -x509 -keyout localhost.pem -out localhost.pem -days 365 -nodes
Now complete the certificate info (remember this isn’t signed by a CA so it will error and prompt on browsers)
Now we can launch a python HTTPS server with the following python script (remeber to change the IP and prot to suit):
import http.server, ssl
server_address = (‘10.10.14.3’, 443)
httpd = http.server.HTTPServer(server_address, http.server.SimpleHTTPRequestHandler)
httpd.socket = ssl.wrap_socket(httpd.socket,
server_side=True,
certfile=’localhost.pem’,
ssl_version=ssl.PROTOCOL_TLSv1_2)
httpd.serve_forever()
and hey presto we have a simple python3 https server running!
It’s using TLS v1_2 as the 1.0 and 1.1 spec will soon be depricated by browsers!